function runProcessor() { $max_fork = 2; $tasks = [ "test1" => "process1", "test2" => "process2", "test3" => "process3", "test4" => "process4", "test5" => "process5", "test6" => "process6", "test7" => "process7", "test8" => "process8", "test9" => "process9", "test10" => "process10", ]; $pids = array(); $pid_count = 0; foreach ( $tasks as $seq => $task ) { $pids[$pid_count] = pcntl_fork(); switch($pids[$pid_count]) { case "0": return execute_task($task); break; case "-1": echo "Unable to fork new process\n"; exit; break; default: $pid_count++; while( count( $pids ) >= $max_fork ) { foreach( $pids as $key=>$pid ) { $pid_status = ""; if( -1 == pcntl_waitpid( $pid, $pid_status, WNOHANG ) ) { unset($pids[$key]); continue; } } usleep(200000); } break; } } exit(); } function execute_task($task_id) { echo "Starting task: ${task_id}\n"; // Simulate doing actual work with sleep(). $execution_time = rand(5, 10); sleep($execution_time); echo "Completed task: ${task_id}. Took ${execution_time} seconds.\n"; exit(); } runProcessor();
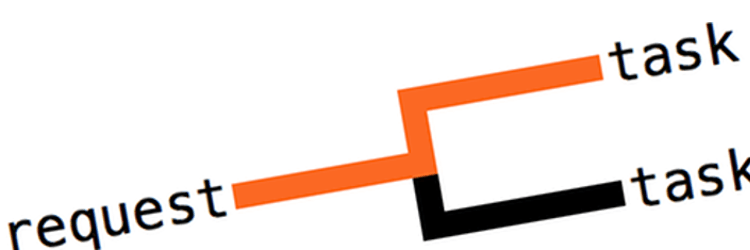